Instruction:
This week’s assignment involves writing a Python program to compute the daily pay for an Uber driver.
The driver gets paid an hourly rate plus a percentage of the miles driven plus the tips provided by passengers. The driver’s daily wage is based on the number of hours worked times his hourly rate. The commission pay is based on the total miles driven times the commission percentage rate. The daily total pay should be computed as the daily wage plus commission pay plus tips.
Write a program that prompts the user for the following input:
- the number of miles driven for the day,
- the number of hours worked for the day
- the amount of tips received for the day
and defines (not prompt) the constant values for:
- the hourly rate for the driver (pick a reasonable value, e.g. $12.50)
- the commission rate (pick a reasonable value e.g. 5%)
Your program should compute and display the output for:
- the daily wage,
- the commission pay
- the tips for the day, and
- the total pay for the day
Your program should include Header comments (what the program does) and in-line comments (the major design steps). Document the values you chose for the cost per paper and percentage rate in your comments as well.
Submit your Python program as a text file (.py) file. In addition, submit a Design outline and a Test plan (3 different test cases) in a Word document or a PDF file which also includes a screenshot of execution of your program for each test case.
Your submission must also adhere to the Submission Requirements document (i.e., Filename and display your name, class, date in the output).
Grading:
10% – Design – outline proper sequence of steps, calculations (if necessary). Identify values of any known constants (e.g. commission rate). Identify what the user inputs will be and what the output will be.
10% – Completeness of your Test plan (at least three test cases). Include screenshots for each test case.
10% – Documentation – Header and in-line comments. Include documentation for the values you chose as the known constants (hourly rate, commission rate) in your comments as well. Documentation of major steps (from Design outline).
70% – Program prompts and executes correctly on all test cases. Satisfies all requirements, compiles, effectiveness and neatness, descriptive variables, def main.
Important note: This is an individual assignment and students are required to submit their original/independent work and adhere to UMGC academic integrity policy. Your submission should adhere to the Submission Requirements document. It is advised that you read all the material, including the Lecture before attempting the assignment. Also, review the example programs and practice exercises. If you do not understand something, reach out to your professor or the UMGC tutor.
- WE OFFER THE BEST CUSTOM PAPER WRITING SERVICES. WE HAVE DONE THIS QUESTION BEFORE, WE CAN ALSO DO IT FOR YOU.
- Assignment status: Writing a Python program to compute the daily pay for an Uber driver
- Already Solved By Our Experts
- (USA, AUS, UK & CA PhD. Writers)
- CLICK HERE TO GET A PROFESSIONAL WRITER TO WORK ON THIS PAPER AND OTHER SIMILAR PAPERS, GET A NON PLAGIARIZED PAPER FROM OUR EXPERTS
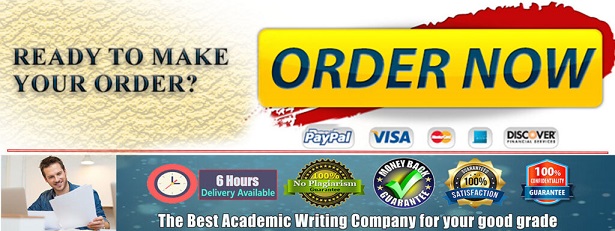